Top 68 Woocommerce Code Hooks and Filters
In this article, you will get to know some hooks and filters for Woocommerce with which you can change and improve your theme. Just copy the code snippets to the functions.php file of your active theme.
WooCommerce support declaration in third party theme
With this code you can add Woocommerce support to any WordPress theme.
function arrow_design_mytheme_add_woocommerce_support() { add_theme_support( 'woocommerce' ); add_theme_support( 'wc-product-gallery-zoom' ); add_theme_support( 'wc-product-gallery-lightbox' ); add_theme_support( 'wc-product-gallery-slider' ); } add_action( 'after_setup_theme', 'arrow_design_mytheme_add_woocommerce_support' );
Adding Custom Currency to WooCommerce
Add this code and change the currency code and symbol according to your requirements and after saving the currency should be findable in the Woocommerce settings.
add_filter( ‘woocommerce_currencies’, ‘arrow_design_add_my_currency’ ); function arrow_design_add_my_currency( $currencies ) { $currencies[‘ABC’] = __( ‘Currency name’, ‘woocommerce’ ); return $currencies; }add_filter(‘woocommerce_currency_symbol’, ‘arrow_design_add_my_currency_symbol’, 10, 2); function arrow_design_add_my_currency_symbol( $currency_symbol, $currency ) { switch( $currency ) { case ‘ABC’: $currency_symbol = ‘$’; break; } return $currency_symbol; }
Show custom billing checkout fields by product id
add_action( 'woocommerce_checkout_fields', 'arrow_design_cutom_checkout_field_conditional_logic' );
function arrow_design_cutom_checkout_field_conditional_logic( $fields ) {foreach( WC()->cart->get_cart() as $cart_item ){ $product_id = $cart_item['product_id'];//change 2020 to your product id if( $product_id == 2020 ) { $fields['billing']['billing_field_' . $product_id] = array( 'label' => __('Custom Field on Checkout for ' . $product_id, 'woocommerce'), 'placeholder' => _x('Custom Field on Checkout for ' . $product_id, 'placeholder', 'woocommerce'), 'required' => false, 'class' => array('form-row-wide'), 'clear' => true ); }}// Return checkout fields. return $fields;}
Hide all shipping method but free shipping
function arrow_design_only_show_free_shipping_when_available( $rates, $package ) { $new_rates = array(); foreach ( $rates as $rate_id => $rate ) { // Only modify rates if free_shipping is present. if ( 'free_shipping' === $rate->method_id ) { $new_rates[ $rate_id ] = $rate; break; } }if ( ! empty( $new_rates ) ) { //Save local pickup if it's present. foreach ( $rates as $rate_id => $rate ) { if ('local_pickup' === $rate->method_id ) { $new_rates[ $rate_id ] = $rate; break; } } return $new_rates; }return $rates; }add_filter( 'woocommerce_package_rates', 'arrow_design_only_show_free_shipping_when_available', 10, 2 );
Replace shop page title
With this code you can easily change the “Shop” heading on the Woocommerce shop page. Just change “My new title” to whatever your title should be.
add_filter( ‘woocommerce_page_title’, ‘arrow_design_shop_page_title’); function arrow_design_shop_page_title($title ) { if(is_shop()) { return “My new title”; } return $title; }
Redirect to the checkout page after clicking add to cart
add_action( 'add_to_cart_redirect', 'arrow_design_add_to_cart_checkout_redirect', 16 ); function arrow_design_add_to_cart_checkout_redirect() { global $woocommerce; $checkout_url = $woocommerce->cart->get_checkout_url(); return $checkout_url; }
Remove product categories from the shop page
This code removes all mentioned product categories from the shop page.
add_action( ‘pre_get_posts’, ‘arrow_design_remove_categories_shop’ ); function arrow_design_remove_categories_shop( $q ) { if ( ! $q->is_main_query() ) return; if ( ! $q->is_post_type_archive() ) return; if ( ! is_admin() && is_shop() && ! is_user_logged_in() ) { $q->set( ‘tax_query’, array(array(‘taxonomy’ => ‘product_cat’,‘field’ => ‘slug’, // Don’t display products in these categories on the shop page ‘terms’ => array( ‘color’, ‘flavor’, ‘spices’, ‘vanilla’ ), ‘operator’ => ‘NOT IN’))); } remove_action( ‘pre_get_posts’, ‘arrow_design_remove_categories_shop’ ); }
Remove the company name from WooCommerce checkout
add_filter( ‘woocommerce_checkout_fields’ , ‘arrow_design_remove_company_name’ );
function arrow_design_remove_company_name( $fields ) { unset($fields[‘billing’][‘billing_company’]); return $fields; }
You can also use the same method to unset other fields:
add_filter( ‘woocommerce_checkout_fields’ , ‘arrow_design_custom_remove_woo_checkout_fields’ ); function arrow_design_custom_remove_woo_checkout_fields( $fields ) {// remove billing fields unset($fields[‘billing’][‘billing_first_name’]); unset($fields[‘billing’][‘billing_last_name’]); unset($fields[‘billing’][‘billing_company’]); unset($fields[‘billing’][‘billing_address_1’]); unset($fields[‘billing’][‘billing_address_2’]); unset($fields[‘billing’][‘billing_city’]); unset($fields[‘billing’][‘billing_postcode’]); unset($fields[‘billing’][‘billing_country’]); unset($fields[‘billing’][‘billing_state’]); unset($fields[‘billing’][‘billing_phone’]); unset($fields[‘billing’][‘billing_email’]); // remove shipping fields unset($fields[‘shipping’][‘shipping_first_name’]); unset($fields[‘shipping’][‘shipping_last_name’]); unset($fields[‘shipping’][‘shipping_company’]); unset($fields[‘shipping’][‘shipping_address_1’]); unset($fields[‘shipping’][‘shipping_address_2’]); unset($fields[‘shipping’][‘shipping_city’]); unset($fields[‘shipping’][‘shipping_postcode’]); unset($fields[‘shipping’][‘shipping_country’]); unset($fields[‘shipping’][‘shipping_state’]); // remove order comment fields unset($fields[‘order’][‘order_comments’]); return $fields; }
Replace “Out of stock” with “sold”
add_filter('woocommerce_get_availability', 'arrow_design_availability_filter_func'); function arrow_design_availability_filter_func($availability) { $availability['availability'] = str_ireplace('Out of stock', 'Sold', $availability['availability']); return $availability; }
Display “product already in cart” instead of “add to cart” button
/** * Change the add to cart text on single product pages */ add_filter( 'woocommerce_product_single_add_to_cart_text', 'arrow_design_woo_custom_cart_button_text' ); function arrow_design_woo_custom_cart_button_text() { global $woocommerce; foreach($woocommerce->cart->get_cart() as $cart_item_key => $values ) { $_product = $values['data']; if( get_the_ID() == $_product->id ) { return __('Already in cart - Add Again?', 'woocommerce'); } } return __('Add to cart', 'woocommerce'); } /** * Change the add to cart text on product archives */ add_filter( 'add_to_cart_text', 'arrow_design_woo_archive_custom_cart_button_text' ); function arrow_design_woo_archive_custom_cart_button_text() { global $woocommerce; foreach($woocommerce->cart->get_cart() as $cart_item_key => $values ) { $_product = $values['data']; if( get_the_ID() == $_product->id ) { return __('Already in cart', 'woocommerce'); } } return __('Add to cart', 'woocommerce'); }
Make billing and shipping fields required
add_filter( 'woocommerce_checkout_fields', 'arrow_design_woo_filter_account_checkout_fields' ); function arrow_design_woo_filter_account_checkout_fields( $fields ) { // Billing fields $fields['billing']['billing_company']['required'] = true; $fields['billing']['billing_email']['required'] = true; $fields['billing']['billing_phone']['required'] = true; $fields['billing']['billing_state']['required'] = true; $fields['billing']['billing_first_name']['required'] = true; $fields['billing']['billing_last_name']['required'] = true; $fields['billing']['billing_address_1']['required'] = true; $fields['billing']['billing_address_2']['required'] = true; $fields['billing']['billing_city']['required'] = true; $fields['billing']['billing_postcode']['required'] = true; // Shipping fields $fields['shipping']['shipping_company']['required'] = true; $fields['shipping']['shipping_phone']['required'] = true; $fields['shipping']['shipping_state']['required'] = true; $fields['shipping']['shipping_first_name']['required'] = true; $fields['shipping']['shipping_last_name'] = true; $fields['shipping']['shipping_address_1'] = true; $fields['shipping']['shipping_address_2'] = true; $fields['shipping']['shipping_city'] = true; $fields['shipping']['shipping_postcode'] = true; return $fields; }
Add a custom field to a product variation
//Display Fields add_action( 'woocommerce_product_after_variable_attributes', 'arrow_design_variable_fields', 10, 2 ); //JS to add fields for new variations add_action( 'woocommerce_product_after_variable_attributes_js', 'variable_fields_js' ); //Save variation fields add_action( 'woocommerce_process_product_meta_variable', 'arrow_design_variable_fields_process', 10, 1 ); function arrow_design_variable_fields( $loop, $variation_data ) { ?> <tr> <td> <div> <label></label> <input type="text" size="5" name="my_custom_field[]" value=""/> </div> </td> </tr> <tr> <td> <div> <label></label> </div> </td> </tr> <?php } function arrow_design_variable_fields_process( $post_id ) { if (isset( $_POST['variable_sku'] ) ) : $variable_sku = $_POST['variable_sku']; $variable_post_id = $_POST['variable_post_id']; $variable_custom_field = $_POST['my_custom_field']; for ( $i = 0; $i < sizeof( $variable_sku ); $i++ ) : $variation_id = (int) $variable_post_id[$i]; if ( isset( $variable_custom_field[$i] ) ) { update_post_meta( $variation_id, '_my_custom_field', stripslashes( $variable_custom_field[$i] ) ); } endfor; endif; }
Show WooCommerce product Categories in a list
function arrowDesign_showProduct_categoriesInAList(){ $args = array( 'number' => $number, 'orderby' => $orderby, 'order' => $order, 'hide_empty' => $hide_empty, 'include' => $ids ); $product_categories = get_terms( 'product_cat', $args ); $count = count($product_categories); if ( $count > 0 ){ echo "<ul>"; foreach ( $product_categories as $product_category ) { echo '<li><a href="' . get_term_link( $product_category ) . '">' . $product_category->name . '</li>'; }//end for each category found echo "</ul>"; }//end if count>0 }//end function arrowDesign_showProduct_categoriesInAList /////testing function by displaying on on the product page, using 'woocommerce_before_main_content' hook // add_action( 'woocommerce_before_main_content', 'arrowDesign_showProduct_categoriesInAList', 20, 0 ); // /////////end, testing function output, on product page
Change “from” email address
function arrow_design_woo_custom_wp_mail_from_name() { $emailNameToSendFrom = "Arrow Design"; return $emailNameToSendFrom; } add_filter( 'wp_mail_from_name', 'arrow_design_woo_custom_wp_mail_from_name', 99 ); function arrow_design_woo_custom_wp_mail_from() { $emailAddressToSendFrom = "cathal@arrowdesign.ie"; return $emailAddressToSendFrom; } add_filter( 'wp_mail_from', 'arrow_design_woo_custom_wp_mail_from', 99 );
Set minimum order amount
add_action( 'woocommerce_checkout_process', 'arrow_design_wc_minimum_order_amount' ); function arrow_design_wc_minimum_order_amount() { global $woocommerce; $minimum = 50; if ( $woocommerce->cart->get_cart_total(); < $minimum ) { $woocommerce->add_error( sprintf( 'You must have an order with a minimum of %s to place your order.' , $minimum ) ); } }
Add Custom Fields To Emails
After adding the code to your functions.php file, follow these steps:
- Change the meta key name
- Add a custom field in the order post (for example key = “Tracking Code” value = abcdefg)
- The next time a user receives an email, this field will be displayed
add_filter(‘woocommerce_email_order_meta_keys’, ‘arrow_design_my_custom_order_meta_keys’);
function arrow_design_my_custom_order_meta_keys( $keys ) { $keys[] = ‘Tracking Code’; // This will look for a custom field called ‘Tracking Code’ and add it to emails return $keys; }
Adding a Custom Field to the Checkout page
This will add a new field after the order notes on the checkout page.
add_action( 'woocommerce_after_order_notes', 'arrow_design_my_custom_checkout_field' );
function arrow_design_my_custom_checkout_field( $checkout ) {echo '<div id="my_custom_checkout_field"><h2>' . __('My Field') . '</h2>';woocommerce_form_field( 'my_field_name', array( 'type' => 'text', 'class' => array('my-field-class form-row-wide'), 'label' => __('Fill in this field'), 'placeholder' => __('Enter something'), ), $checkout->get_value( 'my_field_name' ));echo '</div>';}
After that, the field must be validated when the form is submitted.
add_action('woocommerce_checkout_process', 'arrow_design_my_custom_checkout_field_process');
function arrow_design_my_custom_checkout_field_process() { // Check if set, if its not set add an error. if ( ! $_POST['my_field_name'] ) wc_add_notice( __( 'Please enter something into this new shiny field.' ), 'error' ); }
The last thing to do is to save the field in the custom fields of the order.
add_action( 'woocommerce_checkout_update_order_meta', 'arrow_design_my_custom_checkout_field_update_order_meta' );
function arrow_design_my_custom_checkout_field_update_order_meta( $order_id ) { if ( ! empty( $_POST['my_field_name'] ) ) { update_post_meta( $order_id, 'My Field', sanitize_text_field( $_POST['my_field_name'] ) ); } }
Add Text Before and After Add to Cart
// Before Add to Cart Button: Can be done easily with woocommerce_before_add_to_cart_button hook, example:add_action( ‘woocommerce_before_add_to_cart_button’, ‘arrow_design_before_add_to_cart_btn’ ); function arrow_design_before_add_to_cart_btn(){ echo ‘Some custom text here’; }// After Add to Cart Button: If you are going to add some custom text after “Add to Cart” button, woocommerce_after_add_to_cart_button hook should help you. Example of usage this hookadd_action( ‘woocommerce_after_add_to_cart_button’, ‘arrow_design_after_add_to_cart_btn’ ); function arrow_design_after_add_to_cart_btn(){ echo ‘Some custom text here’; }
Reorder the Checkout Fields in WooCommerce
The fields on the checkout page are separated in four groups:
- Billing — Billing Address
- Shipping — Shipping Address
- Account — Account Login
- Order — Additional information
The fields can be reordered with a special priority parameter. In this example, we want the email field to be displayed at the top, this can be done with this code:
add_filter( ‘woocommerce_checkout_fields’, ‘arrow_design_email_first’ ); function arrow_design_email_first( $checkout_fields ) { $checkout_fields[‘billing’][‘billing_email’][‘priority’] = 4; return $checkout_fields; }
The lowest priority number for the existing fields is 10, so we set it to 4 for the email to make it display at the top. These are the priority numbers for the other fields:
- billing billing_first_name 10
- billing_last_name 20
- billing_company 30
- billing_country 40
- billing_address_1 50
- billing_address_2 60
- billing_city 70
- billing_state 80
- billing_postcode 90
- billing_phone 100
- billing_email 110
Check if a Product Belongs to a Product Category or Tag
if( has_term( 4, ‘product_cat’ ) ) { // do something if current product in the loop is in product category with ID 4 }if( has_term( array( ‘sneakers’, ‘backpacks’ ), ‘product_cat’, 50 ) { // do something if product with ID 50 is either in category “sneakers” or “backpacks” } else { // do something else if it isn’t }if( has_term( 5, ‘product_tag’, 971 ) ) { // do something if product with ID = 971 has tag with ID = 5 }
Change the number of products display in WooCommerce product listing page
/** * Change number of products that are displayed per page (shop page) */ add_filter( 'loop_shop_per_page', 'arrow_design_new_loop_shop_per_page', 20 ); function arrow_design_new_loop_shop_per_page( $cols ) { // $cols contains the current number of products per page based on the value stored on Options -> Reading // Return the number of products you wanna show per page. $cols = 9; return $cols; }
Add custom checkbox fields above the terms and conditions in the WooCommerce checkout
add_action('woocommerce_checkout_before_terms_and_conditions', 'arrow_design_checkout_additional_checkboxes'); function arrow_design_checkout_additional_checkboxes( ){ $checkbox1_text = __( "My first checkbox text", "woocommerce" ); $checkbox2_text = __( "My Second checkbox text", "woocommerce" ); ?> <p class="form-row custom-checkboxes"> <label class="woocommerce-form__label checkbox custom-one"> <input type="checkbox" class="woocommerce-form__input woocommerce-form__input-checkbox input-checkbox" name="custom_one" > <span><?php echo $checkbox1_text; ?></span> <span class="required">*</span> </label> <label class="woocommerce-form__label checkbox custom-two"> <input type="checkbox" class="woocommerce-form__input woocommerce-form__input-checkbox input-checkbox" name="custom_two" > <span><?php echo $checkbox2_text; ?></span> <span class="required">*</span> </label> </p> <?php }add_action('woocommerce_checkout_process', 'arrow_design_custom_checkout_field_process'); function arrow_design_custom_checkout_field_process() { // Check if set, if its not set add an error. if ( ! $_POST['custom_one'] ) wc_add_notice( __( 'You must accept "My first checkbox".' ), 'error' ); if ( ! $_POST['custom_two'] ) wc_add_notice( __( 'You must accept "My second checkbox".' ), 'error' ); }
Extend the admin fields in the WooCommerce orders page
Sometimes it is necessary to expand the admin listing further. In this example, serial numbers needed to be added to the WooCommerce order listing.
- In the “edit_shop_order_columns” function, the fields are rearranged
- In the “shop_order_column” function the fields are managed and the values are provided.
add_filter( 'manage_edit-shop_order_columns', 'arrow_design_edit_shop_order_columns' ) ; function arrow_design_edit_shop_order_columns( $columns ) { $columns = array( 'cb' => '<input type="checkbox" />', 'order_number' => __( 'Order' ), 'order_date' => __( 'Order Date' ), 'order_status' => __( 'Status' ), 'serial' => __( 'Serial Number' ), 'order_total' => __( 'Order Total'), ); return $columns; } add_action( 'manage_shop_order_posts_custom_column', 'arrow_design_shop_order_column', 10, 2);
function arrow_design_shop_order_column( $column, $post_id ) { global $post; $arr = ""; if ( 'serial' === $column ) { echo get_field('order_serial_number',$post_id); } }
add_filter( 'manage_edit-serialnumber_columns', 'arrow_design_edit_serialnumber_columns' );
function arrow_design_edit_serialnumber_columns( $columns ) {$columns = array( 'cb' => '<input type="checkbox" />', 'title' => __( 'Serial Numner' ), 'products' => __( 'Linked Products' ), 'SKU' => __( 'SKU' ), 'status' => __( 'Status'), 'date' => __( 'Added Date' ) );return $columns; }
add_action( 'manage_serialnumber_posts_custom_column', 'arrow_design_realestate_column', 10, 2); function arrow_design_realestate_column( $column, $post_id ) {global $post; $arr = ""; if ( 'products' === $column ) { $productlist = get_field('products',$post_id); foreach($productlist as $row) { $arr[] = get_the_title($row); } echo implode(",",$arr); } $sku = ""; if ( 'SKU' === $column ) { $productlist = get_field('products',$post_id); foreach($productlist as $row) { $product = wc_get_product( $row ); echo $row; $sku[] = $product->get_sku(); } echo implode(",",$sku); }if ( 'status' === $column ) { echo get_field('available',$post_id); }}
Disable the WooCommerce Variable product price range
This code hides the price range and replaces it with the text “From:”.
add_filter( 'woocommerce_variable_price_html', 'arrow_design_variation_price_format_min', 9999, 2 ); function arrow_design_variation_price_format_min( $price, $product ) { $prices = $product->get_variation_prices( true ); $min_price = current( $prices['price'] ); $price = sprintf( __( 'From: %1$s', 'woocommerce' ), wc_price( $min_price ) ); return $price; }
Hide a WooCommerce Category from the Search Result
function arrow_design_hide_rentals_from_search_pre_get_posts( $query ) { if (!is_admin() && $query->is_main_query() && $query->is_search()) { $query->set( ‘post_type’, array( ‘product’ ) );//in the current case i want to hide “rental” category, you can easily replace this with some other product category slug $tax_query = array( array( ‘taxonomy’ => ‘product_cat’, ‘field’ => ‘slug’, ‘terms’ => ‘rentals’, ‘operator’ => ‘NOT IN’, ), );$query->set( ‘tax_query’, $tax_query ); } }add_action( ‘pre_get_posts’, ‘arrow_design_hide_rentals_from_search_pre_get_posts’);
Remove the WooCommerce Checkout fields
add_filter( 'woocommerce_checkout_fields' , 'arrow_design_custom_override_checkout_fields' ); function arrow_design_custom_override_checkout_fields( $fields ) { unset($fields['billing']['billing_first_name']); unset($fields['billing']['billing_last_name']); unset($fields['billing']['billing_company']); unset($fields['billing']['billing_address_1']); unset($fields['billing']['billing_address_2']); unset($fields['billing']['billing_city']); unset($fields['billing']['billing_postcode']); unset($fields['billing']['billing_country']); unset($fields['billing']['billing_state']); unset($fields['billing']['billing_phone']); unset($fields['order']['order_comments']); unset($fields['billing']['billing_email']); unset($fields['account']['account_username']); unset($fields['account']['account_password']); unset($fields['account']['account_password-2']); return $fields; }
If you don’t want to remove all fields, but for example only the address fields, then change the code as follows:
add_filter( 'woocommerce_checkout_fields' , 'arrow_design_custom_override_checkout_fields' ); function arrow_design_custom_override_checkout_fields( $fields ) { unset($fields['billing']['billing_address_1']); unset($fields['billing']['billing_address_2']); unset($fields['billing']['billing_city']); unset($fields['billing']['billing_postcode']); unset($fields['billing']['billing_country']); unset($fields['billing']['billing_state']); return $fields; }
Remove Customer Details from the Order Summary
function arrow_design_removing_customer_details_in_emails( $order, $sent_to_admin, $plain_text, $email ){
$mailer = WC()->mailer();
remove_action( 'woocommerce_email_customer_details', array( $mailer, 'customer_details' ), 10 );
remove_action( 'woocommerce_email_customer_details', array( $mailer, 'email_addresses' ), 20 );
}add_action( 'woocommerce_email_customer_details', 'arrow_design_removing_customer_details_in_emails', 5, 4 );
Check if a user has already paid for a product in WooCommerce
function arrow_design_CheckWhetherUserPaid() { $bought = false; // Set HERE ine the array your specific target product IDs $prod_arr = array( '21', '67' ); // Get all customer orders $customer_orders = get_posts( array( 'numberposts' => -1, 'meta_key' => '_customer_user', 'meta_value' => get_current_user_id(), 'post_type' => 'shop_order', // WC orders post type 'post_status' => 'wc-completed' // Only orders with status “completed” )); foreach ( $customer_orders as $customer_order ) { // Updated compatibility with WooCommerce 3+ $order_id = method_exists( $order, 'get_id' ) ? $order->get_id() : $order->id; $order = wc_get_order( $customer_order ); // Iterating through each current customer products bought in the order foreach ($order->get_items() as $item) { // WC 3+ compatibility if ( version_compare( WC_VERSION, '3.0', '<' ) ) { $product_id = $item['product_id']; } else { $product_id = $item->get_product_id(); } // Your condition related to your 2 specific products Ids if ( in_array( $product_id, $prod_arr ) ) { $bought = true; } } } // return “true” if one the specifics products have been bought before by customer return $bought; }
Activate WooCommerce Holiday/Pause Mode
// Trigger Holiday Mode add_action ('init', 'arrow_design_woocommerce_holiday_mode'); // Disable Cart, Checkout, Add Cart function arrow_design_woocommerce_holiday_mode() { remove_action( 'woocommerce_after_shop_loop_item', 'woocommerce_template_loop_add_to_cart', 10 ); remove_action( 'woocommerce_single_product_summary', 'woocommerce_template_single_add_to_cart', 30 ); remove_action( 'woocommerce_proceed_to_checkout', 'woocommerce_button_proceed_to_checkout', 20 ); remove_action( 'woocommerce_checkout_order_review', 'woocommerce_checkout_payment', 20 ); add_action( 'woocommerce_before_main_content', 'arrow_design_wc_shop_disabled', 5 ); add_action( 'woocommerce_before_cart', 'arrow_design_wc_shop_disabled', 5 ); add_action( 'woocommerce_before_checkout_form', 'arrow_design_wc_shop_disabled', 5 ); } // Show Holiday Notice function arrow_design_wc_shop_disabled() { wc_print_notice( 'Our Online Shop is Closed Today :)', 'error'); }
Deny Checkout if a User Has Pending Orders
add_action('woocommerce_after_checkout_validation', 'arrow_design_deny_checkout_user_pending_orders'); function arrow_design_deny_checkout_user_pending_orders( $posted ) { global $woocommerce; $checkout_email = $posted['billing_email']; $user = get_user_by( 'email', $checkout_email ); if ( ! empty( $user ) ) { $customer_orders = get_posts( array( 'numberposts' => -1, 'meta_key' => '_customer_user', 'meta_value' => $user->ID, 'post_type' => 'shop_order', // WC orders post type 'post_status' => 'wc-pending' // Only orders with status "completed" ) ); foreach ( $customer_orders as $customer_order ) { $count++; } if ( $count > 0 ) { wc_add_notice( 'Sorry, please pay your pending orders first by logging into your account', 'error'); } } }
Change the Autofocus Field at the WooCommerce Checkout
add_filter( 'woocommerce_checkout_fields', 'arrow_design_change_autofocus_checkout_field' ); function arrow_design_change_autofocus_checkout_field( $fields ) { $fields['billing']['billing_first_name']['autofocus'] = false; $fields['billing']['billing_email']['autofocus'] = true; return $fields; }
Show Message After the Country Selection at the Checkout
// Part 1 // Add the message notification and place it over the billing section // The "display:none" hides it by default add_action( 'woocommerce_before_checkout_billing_form', 'arrow_design_echo_notice_shipping' ); function arrow_design_echo_notice_shipping() { echo '<div class="shipping-notice woocommerce-info" style="display:none">Please allow 5-10 business days for delivery after order processing.</div>'; } // Part 2 // Show or hide message based on billing country // The "display:none" hides it by default add_action( 'woocommerce_after_checkout_form', 'arrow_design_show_notice_shipping' ); function arrow_design_show_notice_shipping(){ ?> <script> jQuery(document).ready(function($){ // Set the country code (That will display the message) var countryCode = 'FR'; $('select#billing_country').change(function(){ selectedCountry = $('select#billing_country').val(); if( selectedCountry == countryCode ){ $('.shipping-notice').show(); } else { $('.shipping-notice').hide(); } }); }); </script> <?php }
Disable the Payment Method for a Specific Category
add_filter( 'woocommerce_available_payment_gateways', 'arrow_design_unset_gateway_by_category' ); function arrow_design_unset_gateway_by_category( $available_gateways ) { if ( is_admin() ) return $available_gateways; if ( ! is_checkout() ) return $available_gateways; $unset = false; $category_ids = array( 8, 37 ); //change category id here foreach ( WC()->cart->get_cart_contents() as $key => $values ) { $terms = get_the_terms( $values['product_id'], 'product_cat' ); foreach ( $terms as $term ) { if ( in_array( $term->term_id, $category_ids ) ) { $unset = true; break; } } } if ( $unset == true ) unset( $available_gateways['cheque'] ); return $available_gateways; }
Remove the Checkout Terms & Conditions conditionally in WooCommerce
add_action('woocommerce_checkout_init', 'arrow_design_disable_checkout_terms_and_conditions', 10 ); function arrow_design_disable_checkout_terms_and_conditions(){ remove_action( 'woocommerce_checkout_terms_and_conditions', 'wc_checkout_privacy_policy_text', 20 ); remove_action( 'woocommerce_checkout_terms_and_conditions', 'wc_terms_and_conditions_page_content', 30 ); }
Add Trust Logos to the Checkout Page
add_action( 'woocommerce_review_order_after_submit', 'arrow_design_approved_trust_badges' ); function arrow_design_approved_trust_badges() { echo '<div class="trust-badges"> Add trust badges here </div> <div class="trust-badge-message">The trust badges above were added with a Woocommerce hook.</div>'; }
Show Credit Card Logos on Cart Page
add_action( 'woocommerce_after_cart_totals', 'arrow_design_available_payment_methods' ); function arrow_design_available_payment_methods() { echo '<div class="payment-methods-cart-page"> Add credit card logos here </div> <div class="payment-methods-message">The following payment methods are accepted</div>'; }
Change the Breadcrumb Text on the Woocommerce Pages
In this example, the default “Home” text at the beginning at the breadcrumb text will be changed to “All Products”.
add_filter( 'woocommerce_breadcrumb_defaults', 'arrow_design_change_breadcrumb_home_text' ); function arrow_design_change_breadcrumb_home_text( $defaults ) { // Change the breadcrumb home text from 'Home' to 'All Products' $defaults['home'] = 'All Products'; return $defaults; }
Add a Description to the Shop Page
This will add a text under the “Shop” heading on the Shop Page.
function arrow_design__shop_description() { $description = '<p>This is my shop, I hope you will find what you are looking for!</p>'; echo $description; } add_action('woocommerce_archive_description', 'arrow_design__shop_description');
Add Text to a Specific Product Taxonomy
add_action( 'woocommerce_before_single_product', 'arrow_design_product_notice_function' ); function arrow_design_product_notice_function() { if ( is_product() && has_term( ‘discount-25’,'product_tag' ) ) { echo '<p><strong>This product is reduced by 50% until 06/21/2022!</strong></p>'; } }
Block Purchase from Logged Out Users
add_action( 'woocommerce_before_single_product', 'arrow_design_add_message'); add_filter( 'woocommerce_is_purchasable', 'arrow_design_block_admin_purchase' ); function arrow_design_block_admin_purchase($block) { if ( is_user_logged_in() ):return true; else:return false; endif; } function arrow_design_add_message( ){ if ( !is_user_logged_in() ):echo '<H2 style="margin-bottom:25px; text-align:center; background-color:red; font-weight:bold; color:wheat;">PLEASE LOGIN TO PURCHASE THIS PRODUCT</h2>'; endif; }
Redirect Users to a Specific Product After Login
To do this, you need to know the product ID (respectively the post ID) of the product.
if(!function_exists('arrow_design_redirect_me_after_login')){ function arrow_design_redirect_me_after_login(){ $postId = 1; wp_redirect(get_permalink($postId)); exit(); } add_action('wp_login','arrow_design_redirect_me_after_login',10); }
Add an Admin Message to the WordPress Admin Panel
if(!function_exists('arrow_design_admin_notice_message')){ function arrow_design_admin_notice_message() { ?> <div class="notice notice-warning is-dismissible"> <p>This is my admin message!</p> </div> <?php } add_action( 'admin_notices', 'arrow_design_admin_notice_message' ); }
Change the “Shipping” Text on the Cart Page
This example shows how to change the “Shipping” text to “Shipping & Handling” on the Cart Page in the Cart Totals section.
add_filter('gettext', 'translate_text'); add_filter('ngettext', 'translate_text'); function translate_text($translated) { $translated = str_ireplace('Shipping', 'Shipping & Handling', $translated); return $translated; }
Add a Footer Text to the WordPress Login Page
function arrow_design_login_footer_message() { ?> <p style="border-top: 1px solid #0085ba; margin: 0 auto; width: 320px; padding-top: 10px;">Use your Arrow Design email address to login here.</p> <?php } add_action( 'login_footer', 'arrow_design_login_footer_message' );
Remove Email Details from the Order Summary
function arrow_design_remove_customer_email_details($instance) { //remove `customer_details` hooked function remove_action('woocommerce_email_customer_details', array( $instance, 'customer_details'), 10); } add_action('woocommerce_email', 'arrow_design_remove_customer_email_details');
Add Text Before or After the Woocommerce Price on Shop Pages
//////////function to put text before/after woocommerce price on shop pages -------------->START<----------------------- add_filter( 'woocommerce_get_price_html', 'arrowdesign_customTextWithShopPagePrice' ); function arrowdesign_customTextWithShopPagePrice($price){ $ad_customTextWithPriceWooProd = 'xxxxxxxxx'; //change text in bracket to your preferred text //for text after price $newPriceString = $ad_customTextWithPriceWooProd . $price; $newPriceString = "<h2>". $newPriceString ."</h2>"; //for text before price // return $price . $ad_customTextWithPriceWooProd; //after price // return $ad_customTextWithPriceWooProd . $price; //before price //if the price is zero, or no price set //change XXXXX if( (!isset($price)) or (empty($price)) or (is_null($price)) or ($price=="") or ($price ==0) ){ $price = " XXXXX"; } return $newPriceString; }//end function //////////function to put text before/after woocommerce price on shop pages --------------> END <-----------------------
Show a Text Instead of a Price for Products that are Zero
//show text instead of price, for products that are zero, including variations --start--add_filter( 'woocommerce_get_price_html', 'arrow_design_empty_and_zero_price_display', 20, 2 );
function arrow_design_empty_and_zero_price_display( $price, $product ) {//if you wish to display spcific text
// $blank_price = __('Price not yet set: your text', 'woocommerce');
// $zero_price = __('Free, your text', 'woocommerce');$blank_price = __('YOUR TEXT', 'woocommerce');
$zero_price = __('YOUR TEXT', 'woocommerce');if( ! $product->is_type('variable') ) {
if ( '' === $product->get_price() ) {
return $blank_price;
} else if (0 == $product->get_price() ) {
return $zero_price;
}
}
return $price;
}//show text instead of price, for products that are zero, including variations --end—
Remove or Change the Out of Stock Text
//remove or modify out of stock text --start-- function arrow_design_modify_outOfStockText( $translated_text, $text, $domain ) { switch ( $translated_text ) { case 'This product is currently out of stock and unavailable.' : //if you wish to insert text //$translated_text = __( 'This is my new out of stock text', 'woocommerce' ); //if you wish to remove $translated_text = __( '', 'woocommerce' ); break; } return $translated_text; } add_filter( 'gettext', 'arrow_design_modify_outOfStockText', 20, 3 ); //remove or modify out of stock text --end—
Changing the Minimum/Maximum Quantity for all Woocommerce Products
/* * Changing the minimum quantity to 2 for all the WooCommerce products */ function arrow_design_quantity_input_min_callback( $min, $product ) { $min = 2; return $min; } add_filter( 'woocommerce_quantity_input_min', 'arrow_design_quantity_input_min_callback', 10, 2 ); /* * Changing the maximum quantity to 5 for all the WooCommerce products */ function arrow_design_quantity_input_max_callback( $max, $product ) { $max = 5; return $max; } add_filter( 'woocommerce_quantity_input_max', 'arrow_design_quantity_input_max_callback', 10, 2 );
Add Content to the Woocommerce Customer Processing Order Email
add_action( 'woocommerce_email_before_order_table', 'arrow_design_add_content_specific_email', 20, 4 );function arrow_design_add_content_specific_email( $order, $sent_to_admin, $plain_text, $email ) {
if ( $email->id == 'customer_processing_order' ) {
echo '<h2 class="email-upsell-title">Get 10% off</h2><p class="email-upsell-p">Thank you for making this purchase, when you come back use the code "<strong>Arrow10PercentOff</strong>" for 10% discount.</p>';
}
}
Add Content to the Woocommerce Customer Cancelled Order Email
add_action( 'woocommerce_email_before_order_table', 'arrow_design_add_content_specific_email', 20, 4 ); function arrow_design_add_content_specific_email( $order, $sent_to_admin, $plain_text, $email ) { if ( $email->id == 'cancelled_order' ) { echo '<h2>Contact Us</h2><p>We are sorry that we could not process your order. For more information, please contact us at info@arrowdesign.ie</p>'; } }
Add Content to the Woocommerce Customer Completed Order Email
add_action( 'woocommerce_email_before_order_table', 'arrow_design_add_content_specific_email', 20, 4 ); function arrow_design_add_content_specific_email( $order, $sent_to_admin, $plain_text, $email ) { if ( $email->id == 'customer_completed_order' ) { echo '<h2>Get 5% off on your next order</h2><p>Thank you for choosing our shop! As a thank you, you will receive a 5% discount on your next order with the code "Arrow5PercentOff".</p>'; } }
Add Content to the Woocommerce Customer Refunded Order Email
add_action( 'woocommerce_email_before_order_table', 'arrow_design_add_content_specific_email', 20, 4 );function arrow_design_add_content_specific_email( $order, $sent_to_admin, $plain_text, $email ) {
if ( $email->id == 'customer_refunded_order' ) {
echo '<h2>Give us feedback</h2><p>We hope you were happy with our service despite the return. Please give us a short feedback <a>here</a>.</p>';
}
}
Add Content to the Woocommerce Customer Note Email
add_action( 'woocommerce_email_before_order_table', 'arrow_design_add_content_specific_email', 20, 4 ); function arrow_design_add_content_specific_email( $order, $sent_to_admin, $plain_text, $email ) { if ( $email->id == 'customer_note' ) { echo '<h2>Important!</h2><p>Please read this important order note and check your order details.</p>'; } }
Add Content to the Woocommerce Customer New Order Email
add_action( 'woocommerce_email_before_order_table', 'arrow_design_add_content_specific_email', 20, 4 ); function arrow_design_add_content_specific_email( $order, $sent_to_admin, $plain_text, $email ) { if ( $email->id == 'new_order' ) { echo '<h2>Contact Us</h2><p>Thank you for your order. If you have any questions, please feel free to contact us at info@arrowdesign.ie.</p>'; } }
Add Content to the Woocommerce Customer Reset Password Email
// Add Content to the Woocommerce Customer Reset Password Email --------------- start // add_action ( 'woocommerce_email_header', 'arrow_design_add_content_specific_email_reset', 20, 4 ); function arrow_design_add_content_specific_email_reset( $email_heading, $email ) { if ( $email->id == 'customer_reset_password' ) { echo '<h2>Thanks for contacting us.</h2>'; //large, header text echo'<p>Your message Text</p>'; //your message echo '<br/>'; // this adds a space between your content echo $email_heading; // default wordpress pw reset email content - remove, if needed }//end check 'if' customer_reset_password email }//end function // Add Content to the Woocommerce Customer Reset Password Email --------------- end //
Add Content to the Woocommerce Customer New Account Email
// Add Content to the Woocommerce Customer New Account Email ---------------- start // add_action( 'woocommerce_email_header', 'arrow_design_add_content_specific_email_new_account', 20, 4 ); function arrow_design_add_content_specific_email_new_account( $email_heading, $email ){ if ( $email->id == 'customer_new_account' ) { echo '<h2>Thanks for creating an account</h2>'; //large, header text echo'<p>Your message Text</p>'; //your message echo '<br/>'; // this adds a space between your content echo $email_heading; // default woocommerce new account email - remove, if needed }//end if customer new account email }//end function // Add Content to the Woocommerce Customer New Account Email ------------------ end //
Add Content to the Woocommerce Customer On Hold Order Email
// Add Content to the Woocommerce Customer On Hold Order Email -------------- start // add_action( 'woocommerce_email_header', 'arrow_design_add_content_specific_on_hold', 20, 4 ); function arrow_design_add_content_specific_on_hold( $email_heading, $email ) { if ( $email->id == 'customer_on_hold_order' ) { echo '<h2>Contact Us</h2>'; //large, header text echo '<p>Thanks for using our website, if you have any questions, contact us at info@arrowdesign.ie.</p>'; //your message echo '<br/>'; // this adds a space between your content echo $email_heading; // default woocommerce new account email - remove, if needed }//end if customer on hold order email }//end function // Add Content to the Woocommerce Customer On Hold Order Email -------------- end //
Add Content to the Woocommerce Customer Failed Order Email
// Add Content to the Woocommerce Customer Failed Order Email --------------- start // add_action( 'woocommerce_email_header', 'arrow_design_add_content_specific_failed_order', 20, 4 ); function arrow_design_add_content_specific_failed_order( $email_heading, $email ) { if ( $email->id == 'failed_order' ) { echo '<h2>Contact Us</h2>'; //large, header text echo '<p>Thanks for using our website, if you have any questions, contact us at info@arrowdesign.ie.</p>'; //your message echo '<br/>'; // this adds a space between your content echo $email_heading; // default woocommerce new account email - remove, if needed }//end if customer failed order email }//end function // Add Content to the Woocommerce Customer Failed Order Email --------------- end //
Conclusion
We hope you enjoyed this Woocommerce Code Hooks and Filters post and it helped you improve your woocommerce website. You can find more articles here:
Read another Woocommerce post : ‘Woocommerce Single Product Page Hook Guide‘
For more excellent information on this topic, visit medium.com
Arrow Design, based in Dublin, Ireland, provides quality website design services in Dublin and beyond at affordable prices. If you would like help with implementing the above code, or any wordpress website development project, contact us. We love website design and it shows! We provide custom wordpress plugin development, website design training and lots more.
We do it all, so you don’t have to!
Related Posts
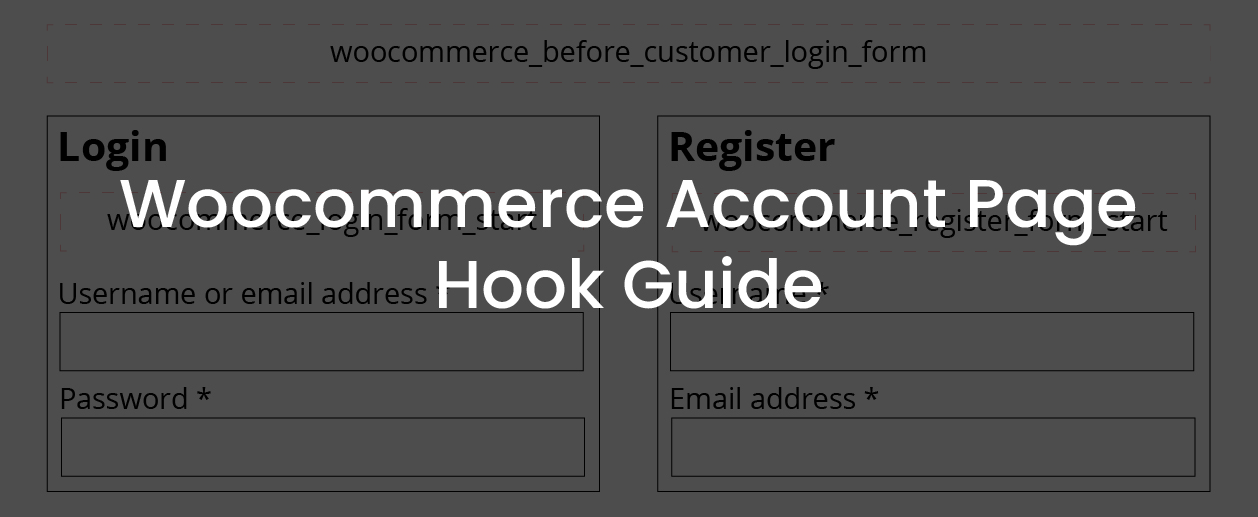
Woocommerce Account Page Hook Guide
In this article you will find a visual hook guide for the Woocommerce Account Pages, like the Login/Register page, the Downloads page or the Orders page.
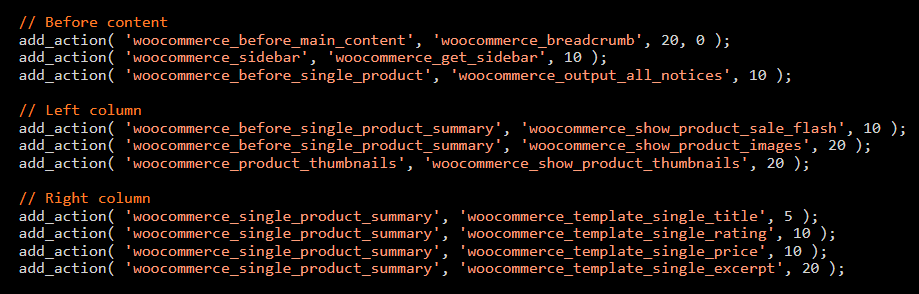
Woocommerce Single Product Page Hook Guide
In this article you will find a visual hook guide for the Woocommerce Single Product Page. This should help you to quickly and easily find the hook positions on the page.
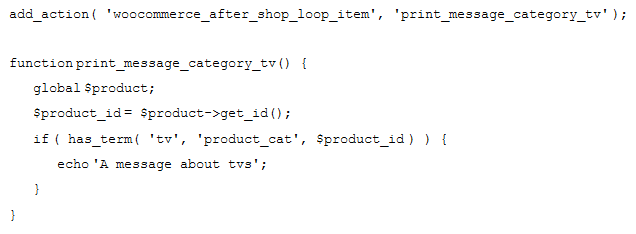
Woocommerce PHP – Product Categories by Product ID
In this tutorial you will learn how to check if a product is assigned to a tag, a category or a custom taxonomy. You can check if a product is is on the shop page, in the cart, in an order & more.
…We do more, so you can do less 🙂