Minimum and Maximum Quantity for Woocommerce Products
In this post we will show you how you can set a minimum and maximum quantity for all Woocommerce products or also how to set it for specific products.
Set the same Quantity for all Products
If you want to have the same fields for minimum and maximum quantities for all products, you can do this with a code snippet. You can find the code here: Top Woocommerce Code Hooks and Filters
Add Fields to the Inventory Tab of the Product Settings
The best place to add the fields for the minimum and maximum quantities is the “Inventory” tab of the “Product data” meta box. There you can add new elements using the woocommerce_product_options_inventory_product_data hook. This code (which is added to the functions.php file) is used to do this:
function arrow_design_qty_add_product_field() { echo '<div class="options_group">'; woocommerce_wp_text_input( array( 'id' => '_wc_min_qty_product', 'label' => __( 'Minimum Quantity', 'woocommerce-max-quantity' ), 'placeholder' => '', 'desc_tip' => 'true', 'description' => __( 'Optional. Set a minimum quantity limit allowed per order. Enter a number, 1 or greater.', 'woocommerce-max-quantity' ) ) ); echo '</div>'; echo '<div class="options_group">'; woocommerce_wp_text_input( array( 'id' => '_wc_max_qty_product', 'label' => __( 'Maximum Quantity', 'woocommerce-max-quantity' ), 'placeholder' => '', 'desc_tip' => 'true', 'description' => __( 'Optional. Set a maximum quantity limit allowed per order. Enter a number, 1 or greater.', 'woocommerce-max-quantity' ) ) ); echo '</div>'; } add_action( 'woocommerce_product_options_inventory_product_data', 'arrow_design_qty_add_product_field' );
function arrow_design_qty_save_product_field( $post_id ) { $val_min = trim( get_post_meta( $post_id, '_wc_min_qty_product', true ) ); $new_min = sanitize_text_field( $_POST['_wc_min_qty_product'] ); $val_max = trim( get_post_meta( $post_id, '_wc_max_qty_product', true ) ); $new_max = sanitize_text_field( $_POST['_wc_max_qty_product'] ); if ( $val_min != $new_min ) { update_post_meta( $post_id, '_wc_min_qty_product', $new_min ); } if ( $val_max != $new_max ) { update_post_meta( $post_id, '_wc_max_qty_product', $new_max ); } } add_action( 'woocommerce_process_product_meta', 'arrow_design_qty_save_product_field' );
If you now enter values in the fields and save them, they will be stored in the meta keys _wc_min_qty_product and _wc_max_qty_product of the post in the table post_meta.
The next step is to add code to fetch the values and dynamically assign the “Minimum Quantity” and “Maximum Quantity” to arguments of quantity selector field. After adding the below code, the quantity selector on the single product page uses the entered values.
function arrow_design_qty_input_args( $args, $product ) { $product_id = $product->get_parent_id() ? $product->get_parent_id() : $product->get_id(); $product_min = arrow_design_get_product_min_limit( $product_id ); $product_max = arrow_design_get_product_max_limit( $product_id ); if ( ! empty( $product_min ) ) { // min is empty if ( false !== $product_min ) { $args['min_value'] = $product_min; } } if ( ! empty( $product_max ) ) { // max is empty if ( false !== $product_max ) { $args['max_value'] = $product_max; } } if ( $product->managing_stock() && ! $product->backorders_allowed() ) { $stock = $product->get_stock_quantity(); $args['max_value'] = min( $stock, $args['max_value'] ); } return $args; } add_filter( 'woocommerce_quantity_input_args', 'arrow_design_qty_input_args', 10, 2 ); function arrow_design_get_product_max_limit( $product_id ) { $qty = get_post_meta( $product_id, '_wc_max_qty_product', true ); if ( empty( $qty ) ) { $limit = false; } else { $limit = (int) $qty; } return $limit; } function arrow_design_get_product_min_limit( $product_id ) { $qty = get_post_meta( $product_id, '_wc_min_qty_product', true ); if ( empty( $qty ) ) { $limit = false; } else { $limit = (int) $qty; } return $limit; }
Now we need to add a validation when the item is added to or updated in the cart.
Validation when Added to the Cart
This needs to be done because it is still possible to add a product with the maximum quantity and then go again to the product and add it a second time.
For validation, we use the woocommerce_add_to_cart_validation filter. In the code below it, if the total quantity of the product in the cart is higher than the maximum quantity set for the product, then the product will not be allowed to be added to the cart and a WooCommerce error message will be displayed.
function arrow_design_qty_add_to_cart_validation( $passed, $product_id, $quantity, $variation_id = '', $variations = '' ) { $product_min = arrow_design_get_product_min_limit( $product_id ); $product_max = arrow_design_get_product_max_limit( $product_id ); if ( ! empty( $product_min ) ) { // min is empty if ( false !== $product_min ) { $new_min = $product_min; } else { // neither max is set, so get out return $passed; } } if ( ! empty( $product_max ) ) { // min is empty if ( false !== $product_max ) { $new_max = $product_max; } else { // neither max is set, so get out return $passed; } } $already_in_cart = arrow_design_qty_get_cart_qty( $product_id );
$product = wc_get_product( $product_id ); $product_title = $product->get_title(); if ( !is_null( $new_max ) && !empty( $already_in_cart ) ) { if ( ( $already_in_cart + $quantity ) > $new_max ) { // oops. too much. $passed = false; wc_add_notice( apply_filters( 'isa_wc_max_qty_error_message_already_had', sprintf( __( 'You can add a maximum of %1$s %2$s\'s to %3$s. You already have %4$s.', 'woocommerce-max-quantity' ), $new_max, $product_title, '<a href="' . esc_url( wc_get_cart_url() ) . '">' . __( 'your cart', 'woocommerce-max-quantity' ) . '</a>', $already_in_cart ), $new_max, $already_in_cart ), 'error' ); } } return $passed; } add_filter( 'woocommerce_add_to_cart_validation', 'arrow_design_qty_add_to_cart_validation', 1, 5 ); /* * Get the total quantity of the product available in the cart. */ function arrow_design_qty_get_cart_qty( $product_id ) { global $woocommerce; $running_qty = 0; // iniializing quantity to 0 // search the cart for the product in and calculate quantity. foreach($woocommerce->cart->get_cart() as $other_cart_item_keys => $values ) { if ( $product_id == $values['product_id'] ) { $running_qty += (int) $values['quantity']; } } return $running_qty; }
Validation on Updating the Cart
This validation must be added because otherwise the customers can change the quantity on the cart page without the restriction being respected. The woocommerce_update_cart_validation filter is used for this.
function arrow_design_qty_get_cart_qty( $product_id , $cart_item_key = '' ) { global $woocommerce; $running_qty = 0; // iniializing quantity to 0 // search the cart for the product in and calculate quantity. foreach($woocommerce->cart->get_cart() as $other_cart_item_keys => $values ) { if ( $product_id == $values['product_id'] ) { if ( $cart_item_key == $other_cart_item_keys ) { continue; } $running_qty += (int) $values['quantity']; } } return $running_qty; } /* * Validate product quantity when cart is UPDATED */ function arrow_design_qty_update_cart_validation( $passed, $cart_item_key, $values, $quantity ) { $product_min = arrow_design_get_product_min_limit( $values['product_id'] ); $product_max = arrow_design_get_product_max_limit( $values['product_id'] ); if ( ! empty( $product_min ) ) { // min is empty if ( false !== $product_min ) { $new_min = $product_min; } else { // neither max is set, so get out return $passed; } } if ( ! empty( $product_max ) ) { // min is empty if ( false !== $product_max ) { $new_max = $product_max; } else { // neither max is set, so get out return $passed; } } $product = arrow_design_get_product( $values['product_id'] ); $already_in_cart = arrow_design_qty_get_cart_qty( $values['product_id'], $cart_item_key ); if ( isset( $new_max) && ( $already_in_cart + $quantity ) > $new_max ) { wc_add_notice( apply_filters( 'wc_qty_error_message', sprintf( __( 'You can add a maximum of %1$s %2$s\'s to %3$s.', 'woocommerce-max-quantity' ), $new_max, $product->get_name(), '<a href="' . esc_url( wc_get_cart_url() ) . '">' . __( 'your cart', 'woocommerce-max-quantity' ) . '</a>'), $new_max ), 'error' ); $passed = false; } if ( isset( $new_min) && ( $already_in_cart + $quantity ) < $new_min ) { wc_add_notice( apply_filters( 'wc_qty_error_message', sprintf( __( 'You should have minimum of %1$s %2$s\'s to %3$s.', 'woocommerce-max-quantity' ), $new_min, $product->get_name(), '<a href="' . esc_url( wc_get_cart_url() ) . '">' . __( 'your cart', 'woocommerce-max-quantity' ) . '</a>'), $new_min ), 'error' ); $passed = false; } return $passed; } add_filter( 'woocommerce_update_cart_validation', 'arrow_design_qty_update_cart_validation', 1, 4 );
Plugins to Set the Minimum and Maximum Quantity
There are some plugins that allow you to set the minimum and maximum quantity for the woocommerce products without coding. These are free plugins that make this possible:
Conclusion
We hope this Minimum and Maximum Quantity for Woocommerce Products was helpful and you can also improve your own website now! You can find more articles here:
Read another Woocommerce post : ‘Adding Custom Currency to WooCommerce‘
Arrow Design, based in Dublin, Ireland, provides quality website design services in Dublin and beyond at affordable prices. If you would like help with implementing the above code, or any wordpress website development project, contact us. We love website design and it shows! We provide custom wordpress plugin development, website design training and lots more.
We do it all, so you don’t have to!
Related Posts
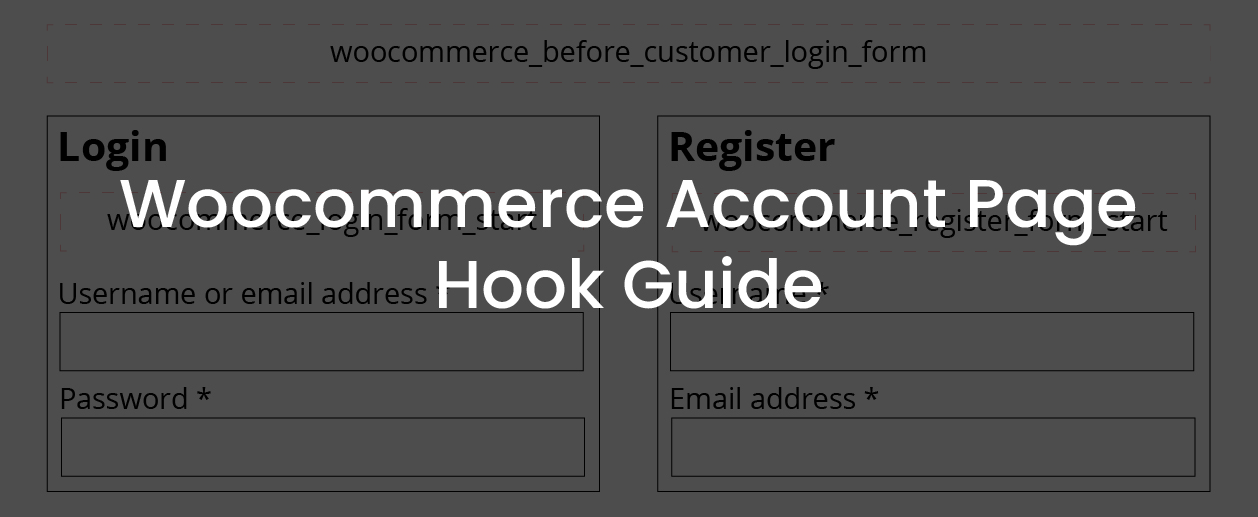
Woocommerce Account Page Hook Guide
In this article you will find a visual hook guide for the Woocommerce Account Pages, like the Login/Register page, the Downloads page or the Orders page.
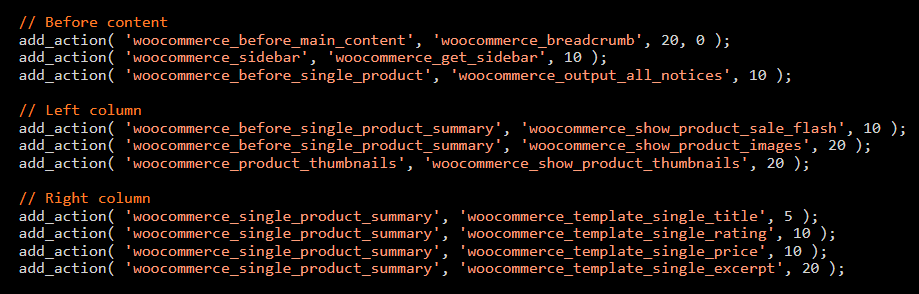
Woocommerce Single Product Page Hook Guide
In this article you will find a visual hook guide for the Woocommerce Single Product Page. This should help you to quickly and easily find the hook positions on the page.
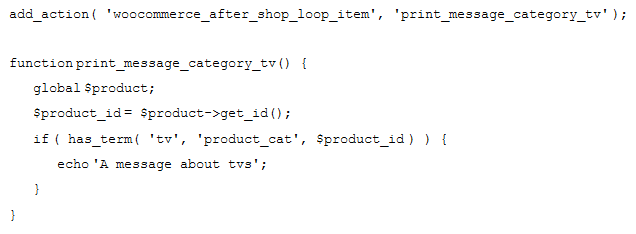
Woocommerce PHP – Product Categories by Product ID
In this tutorial you will learn how to check if a product is assigned to a tag, a category or a custom taxonomy. You can check if a product is is on the shop page, in the cart, in an order & more.
…We do more, so you can do less 🙂